Instructions
You are going to write a program that automatically prints the solution to the FizzBuzz game. These are the rules of the FizzBuzz game:
Your program should print each number from 1 to 100 in turn and include number 100.
When the number is divisible by 3 then instead of printing the number it should print "Fizz".
When the number is divisible by 5, then instead of printing the number it should print "Buzz".`
And if the number is divisible by both 3 and 5 e.g. 15 then instead of the number it should print "FizzBuzz"
e.g. it might start off like this:
1~100 숫자에서 3의 배수만 "Fizz" / 5의 배수만 "Buzz" / 3와 5의 배수면 "FizzBuzz"가 나오도록 하기
# Write your code here 👇
#내가 처음으로 시도한 틀린 코드
for number in range(1, 101) :
if number % 3 == 0:
print("Fizz")
elif number % 5 == 0:
print("Buzz")
elif number % 3 == 0 and number % 5 ==0:
print("FizzBuzz")
else:
print(number)
위 코드가 틀린 이유는
코드 마지막에 number가 3과 5의 배수인지 확인을 하는것인데 위의 세가지 조건 중 하나가 참이 되면 해당 조건의 메시지만 출력하고 그 다음 숫자에 대한 처리는 건너뛰어진다.
그래서 마지막 조건이였던 3과 5의 배수의 숫자에 대한 FIzzBuzz는 출력이 되지 않는 것이다.
마지막 조건을 제일 위로 올려주고 나머지 조건들을 elif에 넣으면 됨.
# Write your code here 👇
for number in range(1, 101):
if number % 3 == 0 and number % 5 == 0:
print("FizzBuzz")
elif number % 3 == 0:
print("Fizz")
elif number % 5 == 0:
print("Buzz")
else:
print(number)
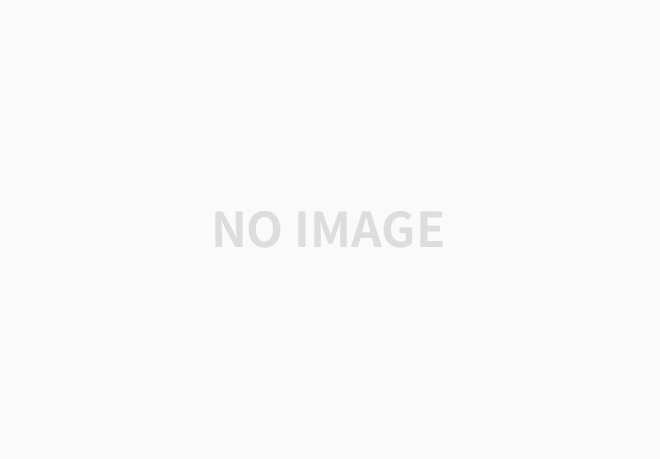
'python' 카테고리의 다른 글
Parameter/ Argument (0) | 2023.11.08 |
---|---|
[Python] ADDING EVEN NUMBERS (0) | 2023.11.02 |
[python]파이썬 for문 반복문 (0) | 2023.11.01 |
[python] BANKER ROULETTE 풀기 (1) | 2023.10.27 |
[python]Heads or Tails 문제 풀기 (0) | 2023.10.27 |